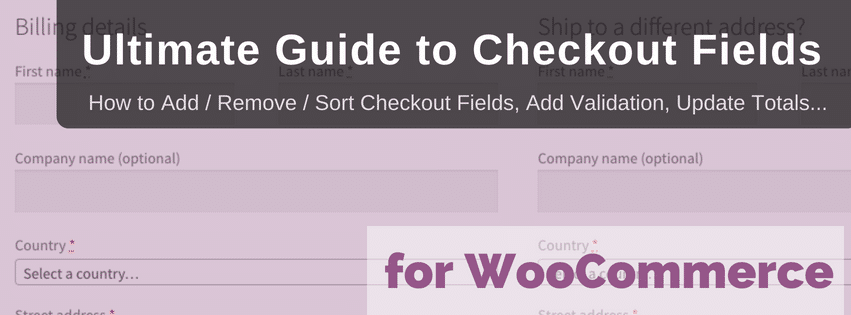
This post is meant as a one stop shop if you’d like to make any kind of customizations to your WooCommerce checkout fields. Whether this is adding additional fields, removing some unneeded ones or changing the order they’re displayed in.
Additionally there will be guides on how do display fields two field side by side, updates the order totals when a field changes and how to add basic field validation.
This is a post with a lot of code snippets and likely requires changes for it to fit your exact needs. Prefer to use a plugin instead? Take a look at my Advanced Checkout Fields for WooCommerce plugin.
Good to Know
These are some good to know files, hooks and functions/methods. Some of these will be used throughout the post, others are related and may be of use for your specific use case.
Files/Functions
includes/class-wc-countries.php
get_default_address_fields()
get_address_fields( $country = '', $type = 'billing_' )
includes/class-wc-checkout.php
get_checkout_fields( $fieldset = '' )
includes/wc-template-functions.php
woocommerce_form_field( $key, $args, $value = null )
Hooks
There are different ways (filters) you can modify checkout fields in WooCommerce. The following hooks can be used accordingly to their use case.
woocommerce_default_address_fields can be used when you’d like to add a address field for all countries.
The woocommerce_billing_fields/woocommerce_shipping_fields hooks are also address only fields, so be aware that these omit the ‘Company’ field for example and is also not the best place to add product fields for example.
woocommerce_checkout_fields is the last in line, this is the very last filter that can be called and contains all the actual checkout fields that will be displayed to the customer.
Checkout Field IDs
When managing the checkout fields you’ll require the checkout field IDs accordingly. Here’s a full list of all the field IDs accordingly.
Billing Fields
- billing_first_name
- billing_last_name
- billing_company
- billing_country
- billing_address_1
- billing_address_2
- billing_city
- billing_state
- billing_postcode
- billing_phone
- billing_email
Shipping Fields
- shipping_first_name
- shipping_last_name
- shipping_company
- shipping_country
- shipping_address_1
- shipping_address_2
- shipping_city
- shipping_state
- shipping_postcode
Account Fields
- account_username
- account_password
Order Fields
- order_comments
Adding Checkout Fields
The following example shows the addition of a basic billing field – using the woocommerce_billing_fields
hook.
/**
* Simple checkout field addition example.
*
* @param array $fields List of existing billing fields.
* @return array List of modified billing fields.
*/
function jeroensormani_add_checkout_fields( $fields ) {
$fields['billing_FIELD_ID'] = array(
'label' => __( 'FIELD LABEL' ),
'type' => 'text',
'class' => array( 'form-row-wide' ),
'priority' => 35,
'required' => true,
);
return $fields;
}
add_filter( 'woocommerce_billing_fields', 'jeroensormani_add_checkout_fields' );
This example adds a very simple text field to the billing fields. There are many additional options you can pass within the array to customize your checkout field. Below is the entire list of the default attributes (and values) that you can pass and override accordingly.
One important thing to note is that the field ID should be prefixed with billing_
, shipping_
, account_
or order_
according to where you’re adding the field to. This ensures the field is automatically processed correctly within WooCommerce Core without additional effort needed.
$defaults = array(
'type' => 'text',
'label' => '',
'description' => '',
'placeholder' => '',
'maxlength' => false,
'required' => false,
'autocomplete' => false,
'id' => $key,
'class' => array(),
'label_class' => array(),
'input_class' => array(),
'return' => false,
'options' => array(),
'custom_attributes' => array(),
'validate' => array(),
'default' => '',
'autofocus' => '',
'priority' => '',
);
That’s are quite a few arguments! but luckily you don’t need to touch most of them as shown with the basic example. Some of the arguments deserve some additional attention;
Type
The type
field is the one that determines what type of field is used. WooCommerce by default has 18 registered field types. Later in this post more about the different checkout field types.
Description
The description
argument adds a description to the front-end and will only be visible when the customer is focussed on the specific field.

Priority
The priority
argument is what determines the order in which the checkout fields will appear. All fields are sorted automatically going from low to high, making it easy to determine the position of a field instead of having to have it at a specific index/position within a list/array.
I’m touching more on sorting checkout fields later.
Validate
The validate
argument is meant to allow for adding additional validity check(s) to the field. Validation checks are done at the checkout when trying to create the order – so only after the customer presses the “Place order” button.
Default available options are; phone
, email
, state
and postcode
. Later in this post I’ll show you can Create a custom checkout field validation.
Checkout Field Types
There are 18 different field types you can choose from by default. I’ll list them all below, some are for more generic use and others are more for one time use such as ‘Country’ or ‘State’, but if wanted you can use them multiple times.
- country
- state
- textarea
- checkbox
- text
- password
- datetime
- datetime-local
- date
- month
- time
- week
- number
- url
- tel
- select
- radio
Creating a Custom Checkout Field Type
Is the field you’re looking for not available in the default list? It is possible to create your own custom field type and use it accordingly. Here’s a quick example of creating a ‘description’ field type that simply adds a description text as a field to the checkout page.
/**
* Simple example of a custom WC checkout field.
*/
function js_description_wc_checkout_field( $field, $key, $args, $value ) {
$sort = $args['priority'] ? $args['priority'] : '';
$container_class = esc_attr( implode( ' ', $args['class'] ) );
$container_id = esc_attr( $args['id'] ) . '_field';
ob_start();
?><p class="form-row <?php echo $container_class; ?>" id="<?php echo $container_id; ?>" data-priority="<?php echo esc_attr( $sort ); ?>">
<?php echo wp_kses_post( $args['label'] ); ?>
</p><?php
return ob_get_clean();
}
add_filter( 'woocommerce_form_field_js_description', 'js_description_wc_checkout_field', 10, 4 );
Since ‘description’ is quite general I’ve prefixed it with js_
to ensure the chances of it conflicting is close to zero. With this code in place you can now add your custom field using the js_description
in the type
parameter. It shows the label
argument as the description. Of course you can modify this to output any custom HTML field you want/need.
Take a look at the woocommerce_form_field()
function to see how the default fields are formatted and structured. This shows a lot of what is expected and how you can create your own custom field type.
Update Totals on Field Change
By default WooCommerce will update the order totals table automatically when the required address fields are all filled out and when any of those fields change. Updating the order totals table when your custom field changes is very easy..
When adding your custom field, simply add a update_totals_on_change
to the class
attribute and it will automagically update the totals whenever the field value changes.
/**
* Update totals
*/
function jeroensormani_add_checkout_fields( $fields ) {
$fields['billing_FIELD_ID'] = array(
'label' => __( 'FIELD LABEL' ),
'type' => 'text',
'class' => array( 'form-row-wide', 'update_totals_on_change' ),
'priority' => 35,
'required' => true,
);
return $fields;
}
add_filter( 'woocommerce_billing_fields', 'jeroensormani_add_checkout_fields' );
Adding Checkout Fields in the Admin
The admin interface does not show the newly added field automatically. There are additional filters where your custom field(s) need to be added to. The following are available for the shipping/billing fields;
woocommerce_admin_billing_fields
woocommerce_admin_shipping_fields
Adding your custom field here is easier then adding it to the checkout, you only have to pass the field ID and the label – most of the time. The following adds the newly added checkout field to the admin.
function js_woocommerce_admin_billing_fields( $fields ) {
$fields['FIELD_ID'] = array(
'label' => __( 'FIELD LABEL' ),
'show' => true,
);
return $fields;
}
add_filter( 'woocommerce_admin_billing_fields', 'js_woocommerce_admin_billing_fields' );
Take note that the FIELD_ID
must be without the billing_ / shipping_
prefix as WooCommerce adds this automatically.
There are additional attributes you can pass to the array. You can already see I passed show => true
in the example. Passing that attribute => value ensures the field and value are shown similar to the Email/Phone fields on the order view.
The types of editing fields are a bit more limited then the checkout fields, you can only choose to make it a text field (default) or set the 'type' => 'select'
to make the edit field a dropdown.
Removing Checkout Fields
Removing any checkout field is quite easy. The only thing to keep in mind is that the ‘Country’ field is a non-removable field as WooCommerce Core does a hard check to see if its available. If you do remove it the order cannot be completed and will give an error saying “Please enter an address to continue.”.
Now – I can’t think of any reason why any store would need this, but the following code removes ALL checkout fields that can be removed, and Woo will still work as expected. You can however easily use this snippet and simply eliminate the code that you don’t want.
/**
* Remove all possible fields - example.
*/
function js_remove_checkout_fields( $fields ) {
// Billing fields
unset( $fields['billing']['billing_company'] );
unset( $fields['billing']['billing_email'] );
unset( $fields['billing']['billing_phone'] );
unset( $fields['billing']['billing_state'] );
unset( $fields['billing']['billing_first_name'] );
unset( $fields['billing']['billing_last_name'] );
unset( $fields['billing']['billing_address_1'] );
unset( $fields['billing']['billing_address_2'] );
unset( $fields['billing']['billing_city'] );
unset( $fields['billing']['billing_postcode'] );
// Shipping fields
unset( $fields['shipping']['shipping_company'] );
unset( $fields['shipping']['shipping_phone'] );
unset( $fields['shipping']['shipping_state'] );
unset( $fields['shipping']['shipping_first_name'] );
unset( $fields['shipping']['shipping_last_name'] );
unset( $fields['shipping']['shipping_address_1'] );
unset( $fields['shipping']['shipping_address_2'] );
unset( $fields['shipping']['shipping_city'] );
unset( $fields['shipping']['shipping_postcode'] );
// Order fields
unset( $fields['order']['order_comments'] );
return $fields;
}
add_filter( 'woocommerce_checkout_fields', 'js_remove_checkout_fields' );
Sorting Checkout Fields
Positioning checkout fields is luckily very easy – mostly. WooCommerce Core does all the heavy lifting in term of actually sorting them, you just provide have to provide the correct priority to the field(s).
Checkout Field Default Priorities
In order to be able to position your field at the right location you of course need the priority of existing fields. This is the full list of all default fields with their priorities;
- First name – 10
- Last name – 20
- Company name – 30
- Country – 40
- Street address – 50
- Apartment, suite, unit etc. (optional) – 60
- Town / City – 70
- State – 80
- Postcode / ZIP – 90
- Phone – 100
- Email – 110
Note: For many countries the priority for the Postcode / ZIP field is changed to 65 by WooCommerce.
Repositioning Existing Checkout Fields
You’ve already seen how to provide the priority
attribute when adding a new checkout field, to re-position a existing field you can do something like below to accomplish that.
/**
* Reposition email/phone fields.
*/
function js_sort_checkout_fields( $fields ) {
$fields['billing']['billing_email']['priority'] = 22;
$fields['billing']['billing_phone']['priority'] = 23;
return $fields;
}
add_filter( 'woocommerce_checkout_fields', 'js_sort_checkout_fields' );
Aligning checkout fields
By default the first- and last name fields are the only two fields that are aligned side by side and the rest of the fields are full width. Aligning the checkout fields can be done by adding one of the below strings to the class
attribute when adding a field.
form-row-first
form-row-last
form-row-wide
The first and last name fields have the form-row-first
and form-row-last
values accordingly to show them side by side. This is an example that gives the same treatment to the two address fields to show them side by side.
function js_align_address_checkout_fields( $fields ) {
if ( ( $key = array_search( 'form-row-wide', $fields['billing_address_1']['class'] ) ) !== false ) {
unset( $fields['billing_address_1']['class'][ $key ] );
}
if ( ( $key = array_search( 'form-row-wide', $fields['billing_address_2']['class'] ) ) !== false ) {
unset( $fields['billing_address_2']['class'][ $key ] );
}
$fields['billing_address_1']['class'][] = 'form-row-first';
$fields['billing_address_2']['class'][] = 'form-row-last';
return $fields;
}
add_filter( 'woocommerce_billing_fields', 'js_align_address_checkout_fields' );

Custom Checkout Field Validation
I’ve touched on checkout field validation shortly before showing the WooCommerce Core validation options. Now I’d like to show a custom validation rule. In my example below I added a validation that checks for a valid 10-alphanumeric customer club number.
/**
* Add custom field validation
*/
function js_custom_checkout_field_validation( $data, $errors ) {
foreach ( WC()->checkout()->get_checkout_fields() as $fieldset_key => $fieldset ) {
foreach ( $fieldset as $key => $field ) {
if ( isset( $field['validate'] ) && in_array( 'club-number', $field['validate'] ) ) {
if ( ! empty( $data[ $key ] ) && ! preg_match( '/[a-z0-9]{10}/', $data[ $key ] ) ) {
$errors->add( 'validation', 'Looks like your club number is invalid.' );
}
}
}
}
}
add_action( 'woocommerce_after_checkout_validation', 'js_custom_checkout_field_validation', 10, 2 );
Here’s a corresponding checkout field snippet that adds the ‘Club number’ field with its validation accordingly.
**
* Add Club number field.
*/
function jeroensormani_add_checkout_fields( $fields ) {
$fields['billing_club_number'] = array(
'type' => 'text',
'label' => __( 'Club number' ),
'description' => __( 'Please enter your club number found on your club card' ),
'priority' => 35,
'validate' => array( 'club-number' ),
);
return $fields;
}
add_filter( 'woocommerce_billing_fields', 'jeroensormani_add_checkout_fields' );
69 thoughts on “Ultimate Guide to WooCommerce Checkout Fields”
Dear Jeroen
Thank you for your nice guide. I have another special question.
Family and Friends for our wedding do log-in with a specific role. So they have after specific view rights. There is still an online-shop and on the checkout-page they can fill out fields about lastname, firstname and e-mail-adress, but in this situation, they overwrite the e-mail-adress for this role. Have you any idea, how it is possible to do, that the e-mail-adress won’t overwritten and the e-mail of ordering an item will be sent?
Thank you and best wishes
Dominik
Hi Dominik,
You could try to remove the email field for that role specifically – I haven’t tried this before, so I can’t say if WooCommerce will allow for it or if its a hard-requirement..
If needed this can be done with my Advanced Checkout Fields plugin using the conditional framework.
Cheers,
Jeroen