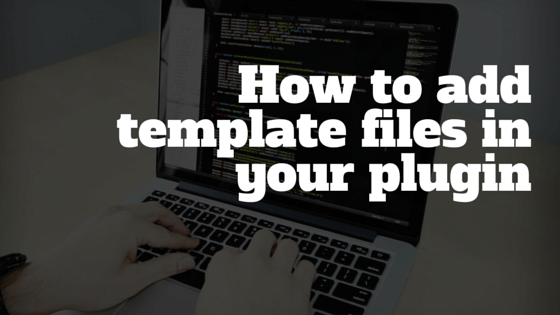
If you’ve worked with WooCommerce before on a website then chances are you’ve also had to copy some of their templates files to your own theme in order to make sure structural changes to the page. This is a great system if you need to output HTML and still want to allow the end-user to be able to change things to fit it to their theme.
Adding template files
For this example I will create a templates
folder within my plugin where I will add a simple page template file called redeem-gift-card.php
. On that page someone can redeem their gift card (to keep line with the previous blog posts about adding custom WooCommerce fields to products where I also used gift cards as an example).
In this example the gift card will be loaded via shortcode, you also could create a custom endpoint, page templates etc if you want to.
Template functions
In order for the templates to work we need to be able to locate the right templates when they are called. With the following code the plugin first look for the called template file in themes/your-theme/woocommerce-plugin-templates/{template-name}
, if that isn’t found it will search in your main theme file themes/your-theme/{template-name}
and falls back to the original plugin templates in plugins/woocommerce-plugin-templates/templates/{template-name}
.
Getting the file
The code above locates and return the path to an existing and valid path that can be loaded and included on the page. In order to actually get the template file there is a extra function. The following code can be used directly in the shortcode function to load the files.
This code will use the wcpt_locate_template()
function to find and return the path to a valid template file, when no valid template file is found and the template file doesn’t exist in the plugins’ template folder, which should never occur, a error will be displayed.
Add a shortcode
Adding a shortcode for this example is quite easy, I’ve used the following code to create the shortcode and display out the template file contents on the page.
Currently the template file is still empty, so it will not display anything on the page. If something went wrong with any part of the code or you mis-typed the template file name you will see a error on the page where you added the [redeem_gift_card]
shortcode (given that you enabled WP_DEBUG).
I included the following code in my plugins/woocommerce-plugin-templates/redeem-gift-card.php
, but let your own imagination go wild here.
A good tip I want to give you is to include a header in the template files with information about the template file, when it was last modified etc. As people will be using your template files, they also need to update these if you are updating them. Therefore it is important to keep track of this and always make notes in your plugin changelog.
Copying the template file to your theme
You are now able to create a template folder called woocommerce-plugin-templates
in your theme folder and create the redeem-gift-card.php
file in there. That will immediately override the template file located in the plugin itself.
Here’s how my theme override looks like;
Bonus: Override WP template files with yours
If you’re adding a custom post type in your plugin for example, and want to include the template files in your plugin as they need specific information you can override the template files WordPress wants to load. In order to do so you can use the following code to override the post
and page
templates (I know, this isn’t a CPT, but its good for the example).
This will override ANY post and page with the contents of the post-override.php
and page-override.php
files if they exist in the templates
folder. For the pages/posts it doesn’t make much sense to override, but as mentioned, this can be very useful for templates for Custom Post Types.
Closing words
You can now get started and add your own templates to your plugins. Don’t forget to make a documentation page about it so your end-users will actually know that its possible 😉 Would be a shame if all the hard work was for nothing!
You can find the full plugin demo plugin here: https://gist.github.com/JeroenSormani/181b8c8a1e5ae6ab8d12 (you still have to create the template folder + files yourself).
I would love to hear from you if this was useful in any way, have feedback, missing something that you’d love to see or just a Thank you 🙂
29 thoughts on “How to add template files in your plugin”
Thank you so much for sharing this information. I was trying to find out proper way for implementing template features in my plugin.
Glad to hear you found it useful 🙂
I am working on wordpress themes development from approx 2 years. Generally custom coding I used inside theme. So from now i am starting to create plugins for custom codes… 🙂
This is exactly what im looking for thankyu so much
Thank you for this writeup. I searched “wordpress plugin template files” and this post came up and it’s exactly what I was looking for. Sure beats looking through woocommerce and other plugins to see how they do it. Appreesh!
Glad it helped you Chris 🙂
Very helpful guide Jeroen thanks for sharing.
I am trying to overwrite my plugin template file with new file I created in my child theme folder. This function below returns empty string.
// Search template file in theme folder.
$template = locate_template( array(
$template_path . $template_name,
$template_name
) );
Any idea why its not showing new template file I have created in child theme folder?
Hi Krupa,
It would require some debugging, which I can’t do for you from here. I’d recommend to check the values of both the variables $template and $template_path in there to see where the function is looking for.
The $template_path is important to have set to the right folder name, thats where its searching for primarily.
Cheers,
Jeroen
Thank you so much for this code. It is so simple to understand and working in a great way as it should. I have only removed “_doing_it_wrong” as it is a private function and not intended to use in themes or plugins. All is well.
Thanks again.
Beautiful turtorial. Clean and simple, and it works, copy and paste, thanks for sharing and spend time contributing to the global scope between jobs!
Thanks for this! Very clear and helpful
Thanks for this usefull tutorial.
I had really enjoyed it.
I had googled for “plugins template wordpress like woocommerce” and found this page.
Did you know any related tuts to learn more about this?
Hi Peyman,
I don’t have any related posts to this, anything you’re missing in this post that I may be able to add?
Cheers,
Jeroen
Hey Jeroen,
Love the tutorial – the first one that i have actually been able to follow and implement(!)
Question, and I am probably missing something fairly obvious here, is it possible to use get_template_part (or something similar) so that I can break down the template that is loaded into sections – I have a number of loops etc. I am attempting to make it even easier for an end user to modify while still maintaining the plugin vs theme functionality this gives me?
Regards
CHRIS
Hi can you please guide how i can get $args values in page?
$this->gaw_get_template( ‘general-stats.php’,array($this,$stats) );
Like this i pass two variables to that template
Never mind i did this but stuck with another issue..
when i call gaw_get_template this function three time its only include first file and not include others one. Means only include one file one time…
return $this->gaw_get_template( ‘general-stats.php’,array(‘current’=>$this,’stats’ =>$stats) );
return $this->gaw_get_template( ‘country-stats.php’,array(‘country_stats’ => $country_stats) );
this example how i include it
Hey never mind i did it too… Thank you so much for this great tutorial.. Its helped alot
Hello, I’ m new in WordPress. How can show this new page from plugin in my site? Thanks
//Thanks a lot. Nice tutorial !!! This works for me…and is so simple 🙂
add_filter( ‘page_template’, ‘wpa3396_page_template’ );
function wpa3396_page_template( $page_template )
{
if ( is_page( ‘my-custom-page-slug’ ) ) {
$page_template = dirname( __FILE__ ) . ‘/custom-page-template.php’;
}
return $page_template;
}
//copy from:
// https://wordpress.stackexchange.com/questions/3396/create-custom-page-templates-with-plugins
good post.thank you
Excellent post, thank you so much for sharing this. Just what I have been looking out for the past couple of days 🙂
Hi Jeroen,
(In perfectly correct Dutch! LOL).
Great article! Any tips on how the get the called template php file to not use the theme? I need to create a page template to generate an invoice and that does not look good if it’s using the header(), footer() and sidebar().
Any tips, please?
Hi Patrick,
I don’t believe the templates should be loading those elements automatically unless its loading in the middle of a page (through a shortcode for example)
You may want to hook into a early hook, output the template, and than prevent any further content from loading.
Cheers,
Jeroen
To the point and handy. Thanks for sharing this.
Hi Jeroen
Thanks for yet another insightful tutorial 🙂
I’m trying to use this to replace emails/customer-completed-order.php without much success. Any ideas? I think I’m getting stuck on how to tweak the wcpt_locate_template function
Thanks
Scott
Hi Scott,
If you’re just looking to replace the default WooCommerce email template I’d recommend overriding it through the standard method by adding it to your child-theme.
Otherwise a different code would be needed to read out the template from a plugin.
Cheers,
Jeroen
Thanks Jeroen – through the theme isn’t an option here since it’s plugin-based functionality at play
I’ve also been tinkering with the woocommerce_locate_template filter without much luck. Back to the drawing board! 🙂
Hi Scott,
That filter should to the trick if you want to do it from a plugin file. Make sure your priority is higher than any other code that may be overriding it through the same filter.
Cheers,
Jeroen